Health Check
When the amount of services increases, it gets more complex to detect and handle whether other services instances are running correctly or not. One solution is that every service should provide a health check with its status and the status of its components.
To create health checks with MicroProfile, we just need to create a class that implements from HealthCheck
and overrides the HealthCheckResponse call()
method. Also, the class requires at least one of the annotations: @Liveness
or @Readiness
. We’ll code the logic that checks that the component of our service is working correctly into the call method.
import javax.enterprise.context.ApplicationScoped; import org.eclipse.microprofile.health.HealthCheck; import org.eclipse.microprofile.health.HealthCheckResponse; import org.eclipse.microprofile.health.HealthCheckResponseBuilder; import org.eclipse.microprofile.health.Liveness; import org.eclipse.microprofile.health.Readiness; @ApplicationScoped @Liveness @Readiness public class DatabaseHealthCheck implements HealthCheck { @Override public HealthCheckResponse call() { HealthCheckResponseBuilder responseBuilder = HealthCheckResponse.named("database"); try { return responseBuilder.up() .withData("name", "my_dummy_db") .build(); }catch(Exception ex){//SqlException return responseBuilder.down().build(); } } }
All our health checks are available at the URL: http://localhost:8080/health
{ "status":"UP", "checks":[ { "name":"database", "status":"UP", "data":{ "Name":"my_dummy_db" }] }
I recommend adding the following dependencies that provide some generic health checks so you can know the memory and thread usage of our application, and to publish a simple dashboard UI with a summary of our health checks. That UI is available at: http://localhost:8080/<app-context>/health-ui/:
<dependency> <groupId>org.microprofile-ext.health-ext</groupId> <artifactId>healthprobe-jvm</artifactId> <version>1.0.5</version> <scope>runtime</scope> </dependency> <dependency> <groupId>org.microprofile-ext.health-ext</groupId> <artifactId>health-ui</artifactId> <version>1.0.5</version> <scope>runtime</scope> </dependency>
http://localhost:8080/stock-service/health-ui/
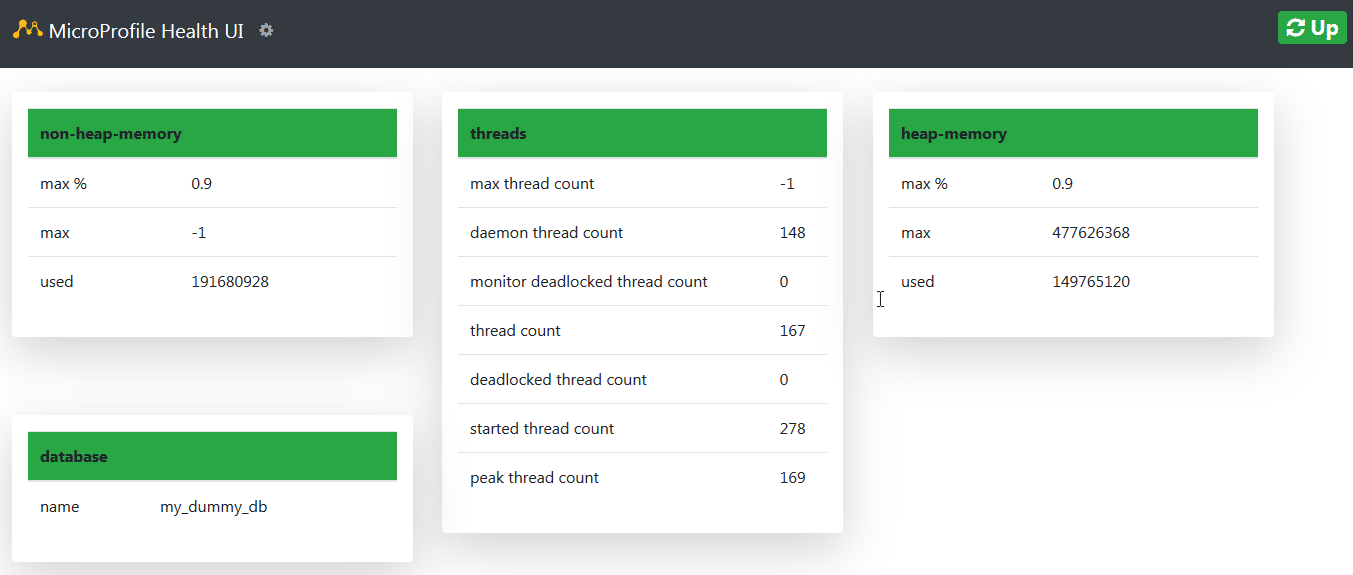