Continued from: MicroProfile overview
Fault Tolerance
This is my favorite MicroProfile module, because it reduces the amount of code and provides really nice features to make our application more resilient, including these fault tolerance policies: Timeout, Retry, Fallback, Bulkhead, and CircuitBreaker.
In the following example, we can see how to use some of them.
@Timeout(value = 5000) @Retry(maxRetries = 3, delay=500) @Fallback(applyOn = CircuitBreakerOpenException.class, fallbackMethod = "getStockFallBack") @CircuitBreaker(successThreshold = 10, requestVolumeThreshold = 4, failureRatio=0.75,delay = 1000) public Stock getStock(String id){ URI apiUri = getURI(); StockClient stockClient = RestClientBuilder.newBuilder() .baseUri(apiUri) .build(StockClient.class); return stockClient.findById(id); } private Stock getStockFallBack(){ return null;//We can return a default, dummy, empty or placeholder result instead of null }
• The @Timeout
annotation prevents waiting forever during the execution of the method.
• The @Retry
annotation will invoke the method up to three times, in case an error occurs, with each invocation having 300ms of delay. This is very useful to recover from a brief network glitch.
• The @CircuitBreaker
annotation prevents repeating invocations of a logic that is failing constantly. For example, the other service might be down. If out of four requests, 75% fail (or three requests fail), then the circuit is open and it will wait for 1000ms to open; then it will be half-open until 10 successful invocations or the circuit is open again. When the circuit is opened, a CircuitBreakerOpenException is thrown.
• The @Fallback
annotation helps us to recover from an exception (unexpected, circuit-breaker, timeout, and more). In the example, we are returning null when the application gets a CircuitBreakerOpenException
.
Now let’s run a failure scenario. If the stock-service is down, because we are using the fault tolerance annotations our application will retry three times. That call won’t exceed five seconds, and after three fails, the circuit breaker is opened, the fallback action is triggered, and we will have a null value as a result.
Our console/logs will look like:
Getting stock for stockId 000001 has failed. The Circuit breaker is open|#] Getting stock for stockId 000002 has failed. The Circuit breaker is open|#] Getting stock for stockId 000003 has failed. The Circuit breaker is open|#]
And the JSON result if we call the list books resource (http://localhost:8080items-service/api/books/) looks like:
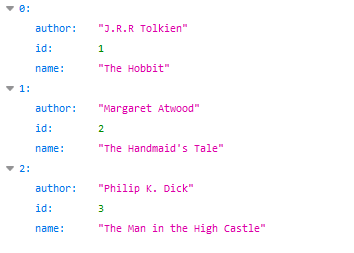
So, our application is resilient enough to handle network issues or even when other required services are down, with a minimum effort and just adding a few annotations.